When you go deep inside C++, you will come across several interesting yet useful concepts!
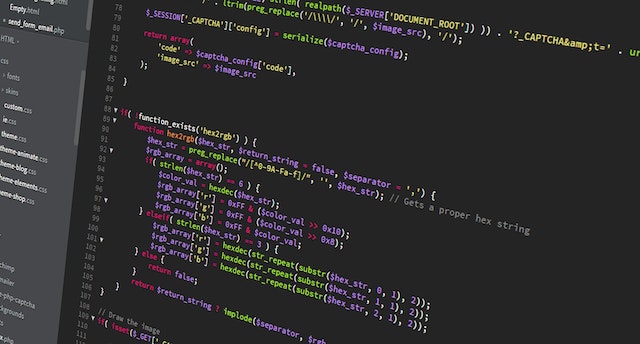
When we talk about the much-crucial array concept, it is quite comprehensive and holds great importance for aspiring programmers.
From the concept of the intersection of an array to sum two arrays to reversing an array, you may come across several topics.
One such versatile topic that we are going to discuss in this tutorial is the intersection of an array in C++.
In the intersection of two arrays, the elements present in one array belong to the elements present in any other array.
There are some wonderful approaches that help in finding the intersection of a given array in the C++ language.
Get ready to learn these approaches in detail with our blog post and learn how to implement them in an efficient way.
Let’s get started!
Intersection of arrays
Array is defined as the derived data type which contains mostly the same kind of data. Arrays generally store values in integer type or float type store the values only in the float type. The derived data type is the one which gets defined by the user only.
In the case of intersection of the two arrays; the set of elements in the A will also belong to that of the B.
Take an example;
Array 1 would be; 1, 2, 3, 4, 5
Array 2 would be 4, 5, 6, 7, 8, 9, 10
The intersection of any two arrays is [ 4, 5]. As 4 and 5 are considered common in both of these elements.
In simpler words, the intersection basically involves the two sets wherein you will get the elements which are present in both the sets.
Problem statement:
In the intersection of two arrays, we can first find all the common elements that are present in the two arrays to create the separate array of all those elements.
The concept would be the same to calculate the intersection set as we do in mathematics. Let us consider, A as the list of certain elements as [ 1,2,3,4] and B being the other list which contains the elements as [ 3, 4, 5, 6]. In this list, the intersection would be [3, 4].
Approaches to find the intersection of two arrays
In order to find the intersection of any two arrays, you can consider the following approaches:
Brute Force Approach
The simple and an easy way to iterate all the items in a first list for all the items in a first list would be to check all the values that are present in a second list.
If that is present, add all its values as the new list by using append () function. The steps you can follow in this case are:
- Create any new empty lists
- For all the elements of any of the list, you can traverse all the elements so as to check all the other elements in a list
- In case any element is present, we can add that element to any other list
In any worst case, these approaches would be having a runtime of the O [m*n] where m and n signifies a certain number of elements in that scenario. Even in the case of any best case, we can still check for all the elements in a list 1 with all the elements in a list 2. The runtime in such a case would be the same.
However, in any worst case, the space needed for an algorithm would either be the O[n] or O[m] as in a new list all the required elements would either be from a list 1 or the list 2. There may be no common elements in this case as the new list will only be having the zero elements. Hence, the space complexity in this case would be the O[1].
Sort list method
Here, the method would be to sort both of the lists and to scan them at the same time. This will help you get the common elements in a given list. For the purpose of sorting, we can do the merge sort.
The steps to follow in this case are as follows:
- Sort both the lists with a merge sort
- Find all the middle points in a given array to divide a list in two parts
- Sort its left part as the middle point
- Also, sort its right part from a middle point
- Scan all the common elements in a list. Create the variables that you can use as an index for each given list. With the help of two variables which are x and y, we can create a new list in order to add all the common elements in it.
As this is the approach of a merge sort, we can take the runtime of the O[nlogn] for both the best and worst case. This can happen as we will divide ‘log n’ numbers to get at the midpoints. At each iteration, we can traverse all the ‘n’ elements in the given list.
The space complexity of merge sort would be the O[n] as well.
When we talk about the space for the second function itself, the space needed for any algorithm would either be O[n] or the O[m]. It means that in the case of a new list, all the elements in the case of list 1 and list 2 would be the same at the intersection level.
Though, in the best possible case, there will not be any type of common element which in the case of a new list would be zero. Hence, the space complexity would be O[1]
Wrapping Up
Learn in-depth about the crucial concepts of intersection of two arrays, reversing an array, sum two arrays and other crucial concepts.
Take the help of this blog post to learn more about how to intersect two arrays in C++. Happy learning!
Leave a Reply